Create and Deploy Simple URL Shortener with AWS CDK and DocumentDB
Create a Simple URL Shortener with AWS CDK and DocumentDB
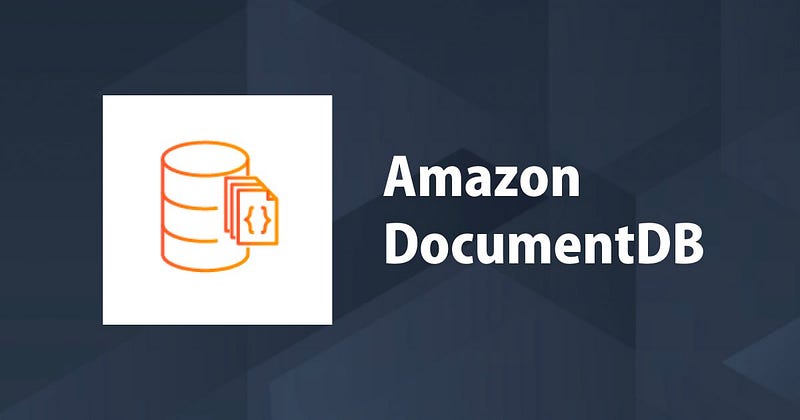
In this tutorial, we will walk you through creating and deploying a simple URL shortener application using AWS CDK, DocumentDB, Lambda and API Gateway. DocumentDB is a document database which is fully compatible with MongoDB 3.6 API. DocumentDB takes lots of inspiration from other AWS Database Services, in which storage and compute layers are separated. This allows fairly simple scalability and high-performance throughput. CDK is a new way to manage AWS resources using common programming languages.
Let’s get started.
In case you haven’t installed AWS CDK yet, here is how to do it.
npm install -g aws-cdk
Let’s build the application logic first
This lambda function will handle requests to API Gateway and create an item in the database with generated short id. We will also save source IP address to the database.
Here we are getting the value from the path parameters and we will query the database searching for that value. If none found we are returning 404 response. If the query returns something, we simply redirect requests to target URL using the API Gateway response.
Since TLS is enabled by default, you will need a public key for interacting with DocumentDB instances.
https://docs.aws.amazon.com/documentdb/latest/developerguide/getting-started.connect.html
Download the key and put it inside src
folder without changing the filename(rds-combined-ca-bundle.pem) or content.
Application logic is all set, let’s move onto provisioning AWS resources
We are creating a VPC with two private and one public subnet, a security group, DocumentDB cluster&instance and two Lambda functions and API gateway REST API resource. We pass the database URL and database name via environment variables to Lambda functions. At the end API Gateway will print out the endpoint for our API Gateway resource. We will also print out the database URL.
Now it’s time for deployment
# Install dependencies
npm install
# Edit .env file for environment variables
vim .env
# Install dependencies and build the source code for Lambda functionscd src && npm i && npm run build
# Deploy using CDK CLI
cd .. && cdk deploy
You should see an output similar to this after a few minutes of provisioning resources.

Now we can try the app logic. We need to do a POST request to /urls API resource. We will get the short version of it afterwards if the request is successful.
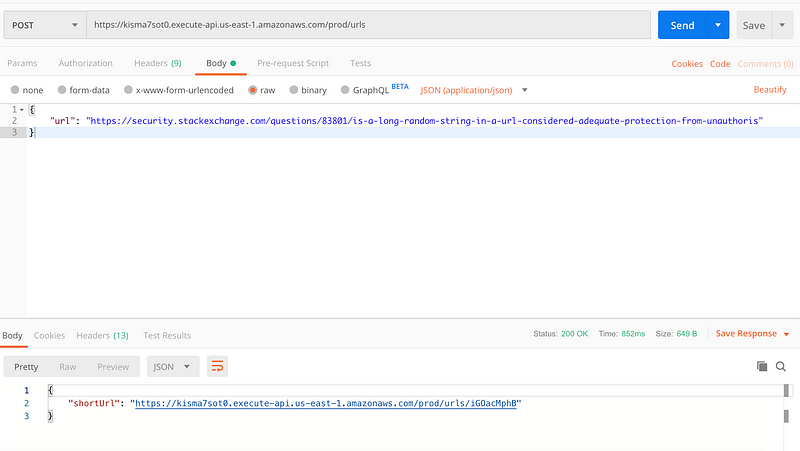
All good, visit the endpoint shortURL via browser and you should be re-directed to StackExchange question.
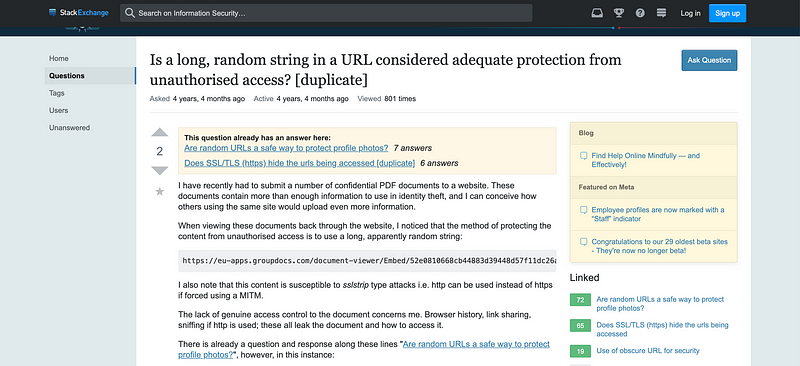
Don’t forget to delete resources after you are done with the application.
cdk destroy
The completed project can be found here: