Cloud Development Kit (CDK) Migration Guide
Cloud Development Kit (CDK) Migration Guide
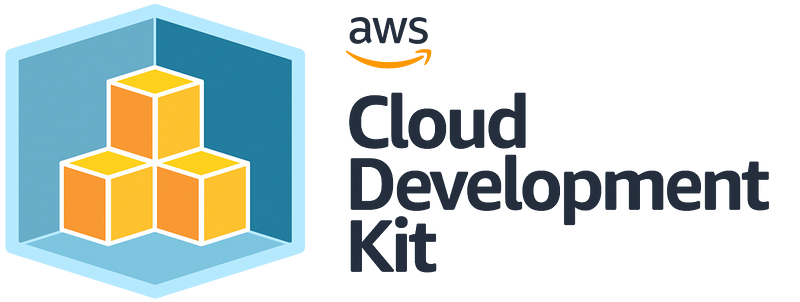
The AWS Cloud Development Kit (CDK) is an open source software development framework from Amazon Web Services (AWS) that allows developers to define cloud infrastructure resources in code using familiar programming languages such as TypeScript, Python, and Java. With the CDK, developers can easily define, provision, and manage AWS resources in a programmatic and scalable way, leveraging the power of AWS CloudFormation under the hood. The CDK simplifies the process of deploying and managing cloud infrastructure, reducing the likelihood of errors and making it easier to maintain infrastructure as code.
The CDK has been released in two major versions, v1 and v2. CDK v1 entered maintenance on June 1, 2022. Support for CDK v1 will end on June 1, 2023.
In this tutorial, I will be showing you to how to migrate an example CDK project from v1 to v2.
You need to update the CDK CLI Toolkit to latest version
npm install -g aws-cdk
Here is the initial project, which was setup with CDK v1.
//index.ts file
import * as cdk from '@aws-cdk/core';
import * as ec2 from '@aws-cdk/aws-ec2';
import { config } from "dotenv";
config();
class EC2BasicsStack extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// Create a new VPC
const vpc = new ec2.Vpc(this, 'VPC');
// Open port 22 for SSH connection from anywhere
const mySecurityGroup = new ec2.SecurityGroup(this, 'SecurityGroup', {
vpc,
securityGroupName: "my-test-sg",
description: 'Allow ssh access to ec2 instances from anywhere',
allowAllOutbound: true
});
mySecurityGroup.addIngressRule(ec2.Peer.anyIpv4(), ec2.Port.tcp(22), 'allow public ssh access')
// We are using the latest AMAZON LINUX AMI
const awsAMI = new ec2.AmazonLinuxImage({generation: ec2.AmazonLinuxGeneration.AMAZON_LINUX_2});
// We define the instance details here
const ec2Instance = new ec2.Instance(this, 'Instance', {
vpc,
instanceType: ec2.InstanceType.of(ec2.InstanceClass.T3, ec2.InstanceSize.NANO),
machineImage: awsAMI,
securityGroup: mySecurityGroup
});
}
}
const app = new cdk.App();
new EC2BasicsStack(app, "EC2BasicsStack", {
env: {
region: process.env.AWS_REGION,
account: process.env.AWS_ACCOUNT_ID
}
});
//package.json file
{
"name": "ec2-basics",
"version": "0.1.0",
"scripts": {
"build": "tsc",
"watch": "tsc -w",
"cdk": "cdk"
},
"devDependencies": {
"@types/node": "8.10.45",
"aws-cdk": "^1.25.0",
"ts-node": "^8.6.2",
"typescript": "^3.8.2"
},
"dependencies": {
"@aws-cdk/aws-ec2": "^1.25.0",
"@aws-cdk/core": "^1.25.0",
"dotenv": "^8.2.0",
"source-map-support": "^0.5.9"
}
}
Main differences between v1 and v2:
- CDK v2 consolidates the stable parts of the AWS Construct Library, including the core library, into a single package, aws-cdk-lib . Before this update, users had to install individual modules separately. Additionally, CDK v2 addresses the challenge of managing multiple packages by eliminating versioning issues.
- Modules that are still in the experimental phase and are being collaboratively developed with the community to create new L2 or L3 constructs are not integrated into aws-cdk-lib. Instead, these experimental modules are distributed as separate packages.
- For stable modules to which new functionality is being added, new APIs (whether entirely new constructs or new methods or properties on an existing construct) receive a Beta1 suffix while work is in progress. (Followed by Beta2, Beta3, and so on when breaking changes are needed.)
- The Construct class, along with its associated types, has been removed from the main CDK library and now exists as a separate library.
- With CDK v2, the environments you deploy into must be bootstrapped using the modern bootstrap stack. The legacy bootstrap stack is no longer supported. CDK v2 furthermore requires a new version of the modern stack. To upgrade your existing environments, re-bootstrap them. It is no longer necessary to set any feature flags or environment variables to use the modern bootstrap stack.
More information can be found here
This is the AWS CDK v2 Developer Guide. The older CDK v1 entered maintenance on June 1, 2022 and will now receive only…docs.aws.amazon.com
Let’s take a look at the updated files to visualize changes.
//index.ts file
import * as cdk from 'aws-cdk-lib';
import * as ec2 from "aws-cdk-lib/aws-ec2";
import { config } from "dotenv";
import { Construct } from 'constructs';
config();
class EC2BasicsStack extends cdk.Stack {
constructor(scope: Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// Create a new VPC
const vpc = new ec2.Vpc(this, 'VPC');
// Open port 22 for SSH connection from anywhere
const mySecurityGroup = new ec2.SecurityGroup(this, 'SecurityGroup', {
vpc,
securityGroupName: "my-test-sg",
description: 'Allow ssh access to ec2 instances from anywhere',
allowAllOutbound: true
});
mySecurityGroup.addIngressRule(ec2.Peer.anyIpv4(), ec2.Port.tcp(22), 'allow public ssh access')
// We are using the latest AMAZON LINUX AMI
const awsAMI = new ec2.AmazonLinuxImage({ generation: ec2.AmazonLinuxGeneration.AMAZON_LINUX_2 });
// We define the instance details here
const ec2Instance = new ec2.Instance(this, 'Instance', {
vpc,
instanceType: ec2.InstanceType.of(ec2.InstanceClass.T3, ec2.InstanceSize.NANO),
machineImage: awsAMI,
securityGroup: mySecurityGroup,
vpcSubnets: {
subnetType: ec2.SubnetType.PUBLIC
},
});
new cdk.CfnOutput(this, "ip-address", {value: ec2Instance.instancePublicIp});
}
}
const app = new cdk.App();
new EC2BasicsStack(app, "EC2BasicsStack", {
env: {
region: process.env.AWS_REGION,
account: process.env.AWS_ACCOUNT_ID
}
});
//package.json file
{
"name": "ec2-basics",
"version": "0.1.0",
"scripts": {
"build": "tsc",
"watch": "tsc -w",
"cdk": "cdk"
},
"devDependencies": {
"aws-cdk": "2.53.0",
"@types/babel__traverse": "7.18.2",
"@types/jest": "^27.5.2",
"@types/node": "10.17.27",
"@types/prettier": "2.6.0",
"jest": "^27.5.1",
"ts-jest": "^27.1.4",
"ts-node": "^10.9.1",
"typescript": "~3.9.7"
},
"dependencies": {
"aws-cdk-lib": "2.53.0",
"constructs": "^10.0.0",
"dotenv": "^8.2.0"
}
}
As you can see, main changes here in this example are
- Packages and versioning changes in `package.json` file
- Changes to Import statements
Please note that certain functionalities that you may have been utilizing in the previous version of CDK may not be accessible in CDK v2. After making the necessary modifications, it is best to carefully review your source code for any potential issues and fix them before deploying your changes.
We need to re-bootstrap our existing environment.
cdk bootstrap
Before deploying your stacks, use cdk diff
to check for unexpected changes to the resources. Changes to logical IDs (causing replacement of resources) are not expected.
cdk diff
According to AWS documentation, it’s normal to see following changes.
- Changes to the
CDKMetadata
resource. - Updated asset hashes.
- Changes related to the new-style stack synthesis. Applies if your app used the legacy stack synthesizer in v1. (CDK v2 does not support the legacy stack synthesizer.)
- The addition of a
CheckBootstrapVersion
rule.
After we are confident with the planned changes, let’s go ahead and deploy our stack.
cdk deploy
It’s recommended that you update your stacks before the end of the current support period, which will end on June 1, 2023.