Manage MongoDB Atlas Deployments with AWS CDK
Manage MongoDB Atlas Deployments with AWS CDK
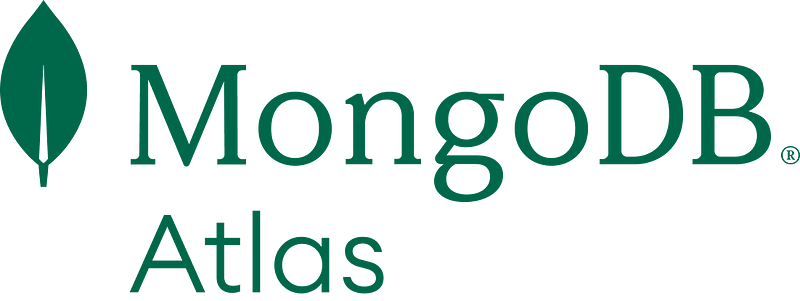
MongoDB Atlas is a fully-managed cloud-based database service offered by MongoDB. It offers a variety of features such as automatic backups, automatic scaling, and easy integration with other cloud services. AWS Cloud Development Kit(CDK) is a tool provided by Amazon Web Services (AWS) that allows you to define infrastructure as code using familiar programming languages such as TypeScript, JavaScript, Python, and others.
MongoDB recently announced general availability for Atlas Integrations for AWS CloudFormation and CDK. In this article, we will go through the process of deploying MongoDB Atlas with AWS CDK.
Prerequisites
Before we start, you will need the following:
- An AWS account
- AWS CDK installed on your local machine
- A MongoDB Atlas account
Step 1: Create a new AWS CDK project
To create a new AWS CDK project, open a terminal or command prompt and run the following command:
cdk init --language=typescript
This command will create a new AWS CDK project in TypeScript language.
Step 2: Install the MongoDB Atlas AWS CDK library
To install the MongoDB Atlas AWS CDK library, run the following command:
npm install @aws-cdk/aws-mongodb-atlas
Step 3: Create a new MongoDB Atlas cluster
To create a new MongoDB Atlas cluster, go to the MongoDB Atlas website and log in to your account. From the dashboard, click the "Build a New Cluster" button. Follow the prompts to set up your new cluster.
Step 4: Retrieve your MongoDB Atlas API keys
To retrieve your MongoDB Atlas API keys, go to the MongoDB Atlas website and log in to your account. From the dashboard, click the "Security" tab, and then click the "API Keys" option. Create a new API key and copy the Public and Private keys.
Step 5: Define the MongoDB Atlas stack in AWS CDK
In your AWS CDK project, create a new file called index.ts
. Add the following code to define the MongoDB Atlas stack:
// index.ts
import * as cdk from "aws-cdk-lib";
import * as atlas from "@mongodbatlas-awscdk/atlas-basic";
import { config } from "dotenv";
import { Construct } from "constructs";
config();
const apiKeys = {
privateKey: process.env.MONGODB_ATLAS_PRIVATE_KEY as string,
publicKey: process.env.MONGODB_ATLAS_PUBLIC_KEY as string,
};
const orgId = process.env.MONGODB_ATLAS_ORGID as string;
const dbPassword = process.env.MONGODB_ATLAS_PASSWORD as string;
const dbUsername = process.env.MONGODB_ATLAS_USERNAME as string;
export class MongoAtlasStack extends cdk.Stack {
constructor(scope: Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
const mongo = new atlas.AtlasBasic(this, "atlas-basic", {
dbUserProps: {
username: dbUsername,
databaseName: "staging",
password: dbPassword,
roles: [
{
roleName: "atlasAdmin",
databaseName: "admin",
},
],
},
clusterProps: {
replicationSpecs: [
{
advancedRegionConfigs: [
{
regionName: "us-east-1",
electableSpecs: {
ebsVolumeType: "STANDARD",
instanceSize: "M10",
nodeCount: 3,
},
},
],
},
],
clusterType: "REPLICASET",
name: "staging-cluster",
},
projectProps: {
name: "staging-project",
orgId,
projectApiKeys: [
{
key: apiKeys.privateKey,
},
{
key: apiKeys.publicKey,
},
],
},
});
}
}
const app = new cdk.App();
new MongoAtlasStack(app, "MongoStack", {
env: {
account: process.env.AWS_ACCOUNT_ID,
region: process.env.AWS_REGION,
},
});
# .env
AWS_ACCOUNT_ID=""
AWS_REGION="us-east-1"
MONGODB_ATLAS_PRIVATE_KEY=""
MONGODB_ATLAS_PUBLIC_KEY=""
MONGODB_ATLAS_ORGID=""
MONGODB_ATLAS_USERNAME""
MONGODB_ATLAS_PASSWORD=""
This code creates a new MongoDB Atlas cluster with the following settings:
- Name:
staging-cluster
- Provider settings:
M10
instance size in theUS_EAST_1
region - Admin user with the password provided in the .env file
- Project:
my-mongo-project
cdk deploy
You can check the MongoDB Atlas Dashboard after the deployment has finished. Don’t forget to remove resources created in this tutorial.
cdk destroy
Are you ready to enhance your AWS Cloud journey? Head over to our website and book a free consultation call.